|
|
|
|
|
 |
|
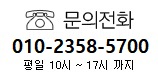 |
|
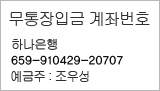 |
|
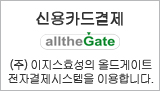 |
|
6만원 이상 무료배송
|
|
주문하시는
총상품금액의 합계가
6만원 이상일 경우
택배비가 무료입니다.
|
|
|
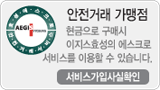 |
|
|
|
|
|
|
작성자:
어라
작성일: 2009-07-07 09:48
조회: 36197
댓글: 0
|
|
|
package test;
import java.util.ArrayList; import java.util.List;
import org.apache.commons.httpclient.HttpClient; import org.apache.commons.httpclient.HttpMethod; import org.apache.commons.httpclient.HttpStatus; import org.apache.commons.httpclient.MultiThreadedHttpConnectionManager; import org.apache.commons.httpclient.NameValuePair; import org.apache.commons.httpclient.methods.GetMethod; import org.apache.commons.httpclient.methods.MultipartPostMethod; import org.apache.commons.httpclient.methods.PostMethod; import org.apache.commons.httpclient.util.EncodingUtil;
public class HttpClientHelper { public HttpClientHelper(String urlStr){ this.urlStr = urlStr; paramList = new ArrayList(); }
private String urlStr = ""; private String content = ""; private int methodType = 0; private int iGetResultCode = 0; private int connectionMaxTime = 5000;
private static final int GETTYPE = 0; private static final int POSTTYPE = 1; private static final int MULTIPARTTYPE = 2;
public String getContent() { return content; }
public int getIGetResultCode() { return iGetResultCode; }
private List paramList = null;
public void setParam(String key, String value) { paramList.add(new NameValuePair(key,value)); }
public int getMethodType() { return methodType; }
public void setMethodType(int methodType) { this.methodType = methodType; }
public int execute() { iGetResultCode = 0; HttpClient client = null; HttpMethod method = null; NameValuePair[] paramArray = new NameValuePair[paramList.size()]; paramList.toArray(paramArray); try { client = new HttpClient(new MultiThreadedHttpConnectionManager()); client.setTimeout(getConnectionMaxTime());
if(methodType == GETTYPE){ GetMethod getMethod = new GetMethod(urlStr); if(paramArray.length > 0) getMethod.setQueryString(EncodingUtil.formUrlEncode(paramArray,"euc-kr")); method = getMethod; }else if(methodType == POSTTYPE){ PostMethod postMethod = new PostMethod(urlStr); for(int k = 0; k < paramArray.length; k++){ postMethod.addParameter(paramArray[k].getName(), new String(paramArray[k].getValue().getBytes(),"ISO-8859-1")); } method = postMethod; }else if(methodType == MULTIPARTTYPE){ MultipartPostMethod multipartPostMethod = new MultipartPostMethod(urlStr); for(int k = 0; k < paramArray.length; k++){ multipartPostMethod.addParameter(paramArray[k].getName(), paramArray[k].getValue()); } method = multipartPostMethod; } // method.setFollowRedirects(true);
iGetResultCode = client.executeMethod(method); if(iGetResultCode == HttpStatus.SC_OK) { content = method.getResponseBodyAsString(); } } catch (Exception e) { iGetResultCode = 0; }finally{ if(method != null) method.releaseConnection(); } return iGetResultCode; }
public int getConnectionMaxTime() { return connectionMaxTime; }
public void setConnectionMaxTime(int connectionMaxTime) { this.connectionMaxTime = connectionMaxTime; } }
--------------------------------------------------------------------------------
package test;
import gshs.eshop.common.httputil.HttpClientHelper;
public class TestSimple { private void testPostMethod() { System.out.println("testPostMethod() start ###################################");
String urlStr = "http://kkaok.pe.kr/frameset/door.jsp"; HttpClientHelper test = new HttpClientHelper(urlStr); // 넘겨줄 파라미터 세팅 test.setParam("act", "/servlet/KBoard?tableName=kjsp"); // setMethodType을 지정하지 않으면 default = 0, (0:get, 1:post, 2:multipart) test.setMethodType(1); // connection 연결시간 설정 default = 5000; test.setConnectionMaxTime(5000);
int rtnCode = test.execute(); // 실행하기 System.out.println(rtnCode); // 결과 값. 200이면 정상 System.out.println(test.getIGetResultCode()); // rtnCode 값을 결과 값이다. System.out.println(test.getContent()); // 해당 페이지의 내용 불러오기 }
private void testGetMethod() { System.out.println("testGetMethod() start ###################################"); String urlStr = "http://kkaok.pe.kr/frameset/door.jsp"; HttpClientHelper test = new HttpClientHelper(urlStr); test.setParam("act", "/servlet/KBoard?tableName=kjsp"); // setMethodType을 지정하지 않으면 default = 0, (0:get, 1:post, 2:multipart) test.setMethodType(0); // connection 연결시간 설정 default = 5000; test.setConnectionMaxTime(5000);
int rtnCode = test.execute(); System.out.println(rtnCode); // 결과 값. 200이면 정상 System.out.println(test.getIGetResultCode()); // rtnCode 값을 결과 값이다. System.out.println(test.getContent()); // 해당 페이지의 내용 불러오기 }
private void testGetMethodSample() { System.out.println("testGetMethodSample() start ###################################"); String urlStr = "http://kkaok.pe.kr/frameset/door.jsp"; HttpClientHelper test = new HttpClientHelper(urlStr); test.setParam("act", "/servlet/KBoard?tableName=kjsp"); // setMethodType을 지정하지 않으면 default = 0, (0:get, 1:post, 2:multipart) test.setMethodType(0); // connection 연결시간 설정 default = 5000; test.setConnectionMaxTime(5000);
int count = 5; int rtnCode = 0; for (int i = 0; i < count; i++) { System.out.println("count : " + (i + 1)); rtnCode = test.execute(); if (rtnCode == 200) { break; } } if (rtnCode == 200) { System.out.println(test.getIGetResultCode()); System.out.println(test.getContent()); } }
private void testNoMethodOnlyUrl() { System.out.println("testNoMethodOnlyUrl() start ###################################"); String urlStr = "http://kkaok.pe.kr/frameset/door.jsp?act=/servlet/KBoard?tableName=kjsp"; HttpClientHelper test = new HttpClientHelper(urlStr); int rtnCode = test.execute(); System.out.println(rtnCode); // 결과 값. 200이면 정상 System.out.println(test.getIGetResultCode()); // rtnCode 값을 결과 값이다. System.out.println(test.getContent()); // 해당 페이지의 내용 불러오기
}
public static void main(String[] args) { TestSimple test = new TestSimple(); test.testGetMethod(); test.testPostMethod(); test.testNoMethodOnlyUrl(); test.testGetMethodSample(); } }
|
|
|
|
|
* 관련 댓글 한말씀 부탁합니다.
|
|
|